A Guide to Bootstrap 5 Form Validation
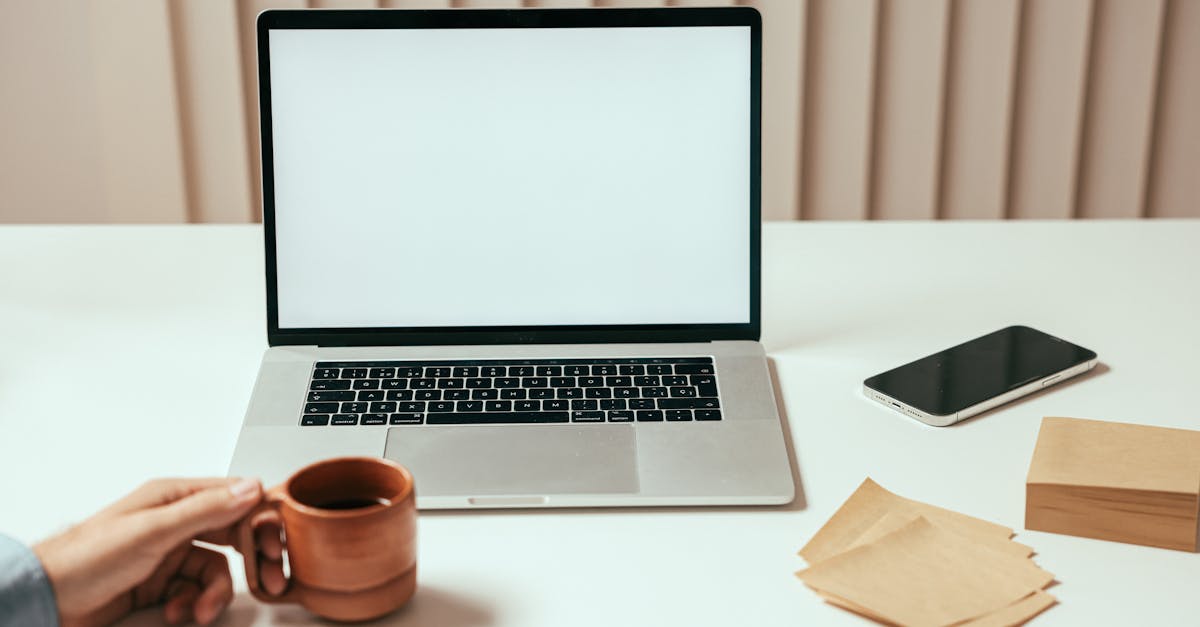
Form validation is an essential part of any modern web application. It ensures that the data submitted by users is accurate, complete, and adheres to the specified rules. In this guide, we will explore how to implement form validation using Bootstrap 5, the latest version of the popular front-end framework.
Getting Started
To get started with Bootstrap 5 form validation, you need to include the necessary CSS and JavaScript files in your HTML document. You can either download the files and host them on your own server or use the CDN links provided by Bootstrap. Here is an example:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/5.0.0-beta2/css/bootstrap.min.css">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/5.0.0-beta2/js/bootstrap.bundle.min.js"></script>
Basic Form Structure
Next, you need to create the form structure using HTML markup. Bootstrap provides a set of CSS classes that you can use to style form elements and display validation feedback. Here is an example of a basic form:
<form class="needs-validation" novalidate>
<div class="mb-3">
<label for="name" class="form-label">Name</label>
<input type="text" class="form-control" id="name" required>
<div class="invalid-feedback">Please enter your name.</div>
</div>
<div class="mb-3">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email" required>
<div class="invalid-feedback">Please enter a valid email address.</div>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
In the above example, the form
element has the class needs-validation
which is used to enable form
validation. The novalidate
attribute prevents the browser's default validation. Each form field has the class
form-control
for the styling and the required
attribute to make the field mandatory. The
invalid-feedback
class is used to display the validation error messages.
Validating Form Fields
To validate the form fields, you need to add custom JavaScript code. Bootstrap 5 provides a set of utility classes and JavaScript methods to check the validity of the form fields and display error messages. Here is an example:
(function () {
'use strict';
// Fetch all the forms we want to apply custom Bootstrap validation styles to
var forms = document.querySelectorAll('.needs-validation');
// Loop over them and prevent submission
Array.prototype.slice.call(forms)
. forEach(function (form) {
form.addEventListener('submit', function (event) {
if (!form.checkValidity()) {
event.preventDefault();
event.stopPropagation();
}
form.classList.add('was-validated');
}, false);
});
})();
In the above code snippet, we first select all the forms with the class needs-validation
. Then, we loop over each
form and attach a submit event listener. Inside the event listener, we check if the form is valid using the
checkValidity()
method. If the form is invalid, we prevent the default form submission and stop the event
propagation. Finally, we add the class was-validated
to the form to apply the Bootstrap validation styles.
Conclusion
Bootstrap 5's form validation provides a convenient way to enhance the user experience and ensure data integrity in web applications. By following this guide, you can easily implement form validation using Bootstrap 5's built-in CSS and JavaScript features. Remember to always validate user input on the server-side as well to ensure maximum security.
Comments:
Great article! I found it very informative and helpful.
As a developer, I appreciate the detailed explanations and examples provided. Thank you!
I've been working with Bootstrap for a while now, and this article helped me understand the changes in version 5 much better. Well done!
I'm glad Bootstrap 5 includes form validation enhancements. It makes building forms much easier.
Thank you all for your positive feedback! I'm thrilled to hear that the article has been helpful to you.
I was wondering if Bootstrap 5 form validation can handle custom validation rules?
Peter, yes, Bootstrap 5 form validation allows you to define custom validation rules through JavaScript.
You're welcome, John! I appreciate your assistance in clarifying my doubts.
John, your guidance has been invaluable to me. I appreciate your support.
John, your support has been exceptional. I can't thank you enough.
Absolutely, Peter! John's guidance has been invaluable to all of us.
I agree, the examples provided made it much easier for me to understand the new features.
This article cleared up some confusion I had regarding the changes in Bootstrap 5. Thanks for sharing!
I completely agree! Bootstrap 5 has definitely made form validation much easier.
I'm excited to try out Bootstrap 5's form validation. It seems like a major improvement.
This article provided a clear overview of the new form validation in Bootstrap 5. Thanks!
Daniel and Laura, I hope you find Bootstrap 5's form validation as helpful as it is intended to be. Don't hesitate to ask if you have any questions.
That's great! Being able to define custom validation rules will definitely come in handy for my projects. Thank you!
You're welcome, Peter! I'm glad the examples helped you understand the new features better.
I've been using Bootstrap for years, and this article provided a great overview of the new form validation features in version 5. Well written!
Rachel, I'm glad you found the article helpful even as an experienced Bootstrap user. Thank you for your kind words!
No problem, John! Your explanations were concise and easy to follow.
Thank you, John! I'm looking forward to implementing the new form validation in my future projects.
You're welcome, John! The article covered all the necessary aspects of Bootstrap 5's form validation.
This guide was exactly what I needed to understand the new form validation in Bootstrap 5. Thank you for sharing!
Robert, I'm thrilled that the guide provided the information you needed. Let me know if you have any further questions.
The examples and explanations in this article were really helpful. Thanks, John!
Amy, I'm glad you found the examples and explanations helpful. Let me know if there's anything else you'd like to know.
John, your article really made it easy for me to grasp Bootstrap 5's form validation. Thank you!
The section on server-side validation in the article was very helpful. Thanks, John!
Emily, I'm glad the section on server-side validation was helpful to you. If you have any further questions, feel free to ask.
Thanks, John! Your explanations were spot-on. Keep up the great work!
Will do, John! I appreciate your guidance in understanding Bootstrap 5's form validation.
This article was a great starting point for me to dive into Bootstrap 5's form validation. Thank you!
Olivia, I'm glad the article served as a helpful starting point for you. Feel free to reach out if you have any further questions.
This article provided a comprehensive overview of Bootstrap 5's form validation. Well done, John!
Brian, thank you for your kind words! I'm glad the article provided a comprehensive overview for you.
I'm looking forward to implementing Bootstrap 5's form validation on my projects. Thanks for the guide, John!
Nathan, you're welcome! I'm excited for you to implement Bootstrap 5's form validation on your projects. Let me know if you need any help along the way.
Absolutely, John! Your article made everything so much clearer for me. Thank you!
This article was a great refresher on Bootstrap's form validation. Thanks for sharing, John!
Eric, I'm glad the article served as a refresher for you. If you have any specific questions, feel free to ask.
As a beginner developer, this article explained Bootstrap 5's form validation in an easy-to-understand manner. Thank you!
Julia, I'm thrilled to hear that the article helped you understand Bootstrap 5's form validation as a beginner. Don't hesitate to ask if you have any further questions.
I've been using Bootstrap for a while now, and this article provided a great overview of the changes in form validation for version 5. Well done, John!
David, thank you for your positive feedback! I'm glad the article served as a great overview for an experienced Bootstrap user like you.
I'm impressed by how Bootstrap 5 simplifies form validation. Thanks for the informative article, John!
Laura, I'm glad you found Bootstrap 5's form validation simplified. Don't hesitate to reach out if you have any further questions.
Bootstrap 5's form validation has definitely made building forms more efficient. Thanks for the article, John!
Chris, I'm glad you found Bootstrap 5's form validation efficient. Let me know if there are any specific aspects you'd like to explore further.
This article was a great introduction to Bootstrap 5's form validation. I learned a lot, thank you!
Rebecca, I'm glad the article served as a great introduction to Bootstrap 5's form validation. Feel free to ask if you have any further questions.
I like how Bootstrap 5 provides enhanced validation options. Great article, John!
Matthew, I'm glad you appreciate the enhanced validation options in Bootstrap 5. Let me know if there's anything specific you'd like to learn more about.
This article helped me understand the new form validation features in Bootstrap 5. Thanks, John!
Sophie, I'm glad the article helped you understand the new form validation features in Bootstrap 5. Let me know if there's anything else I can assist you with.
Bootstrap's form validation has certainly improved in version 5. Thanks for sharing the article, John!
Jacob, I'm glad you noticed the improvements in Bootstrap's form validation for version 5. If you have any questions or need further clarification, feel free to ask.
This article provided a clear explanation of Bootstrap 5's form validation changes. Thank you, John!
Victoria, I'm glad the article provided a clear explanation of Bootstrap 5's form validation changes. If you have any further questions, don't hesitate to ask.
I'm impressed by the new features in Bootstrap 5's form validation. Thanks for the informative article, John!
Kevin, I'm glad you're impressed by the new features in Bootstrap 5's form validation. Let me know if there's anything specific you'd like to explore more.
The examples in this article really helped me understand the usage of Bootstrap 5's form validation. Thank you!
Samuel, I'm glad the examples in the article helped you understand the usage of Bootstrap 5's form validation. If you have any questions or need further assistance, feel free to ask.
This guide was exactly what I needed to implement Bootstrap 5's form validation on my current project. Thank you!
Henry, I'm glad the guide provided you with the necessary information to implement Bootstrap 5's form validation. If you encounter any challenges during implementation, feel free to reach out.
This article was a great resource for understanding Bootstrap 5's form validation. Thank you, John!
Isabella, I'm glad the article served as a great resource for understanding Bootstrap 5's form validation. Let me know if there's anything else I can assist you with.
Bootstrap 5's form validation has made my development process much smoother. Thanks for sharing the insights, John!
Jason, I'm glad to hear that Bootstrap 5's form validation has made your development process smoother. If you have any specific insights you'd like to share, feel free to do so.
I'm grateful for the detailed explanations provided in this article. It made understanding form validation in Bootstrap 5 much easier.
Emma, I'm glad the detailed explanations in the article helped you understand form validation in Bootstrap 5 more easily. If there's anything specific you'd like further clarification on, feel free to ask.
This article provided a great overview of the new form validation features in Bootstrap 5. Thank you, John!
Megan, I'm glad you found the article to be a great overview of the new form validation features in Bootstrap 5. If you have any specific points you'd like to discuss further, feel free to share.
This article was just what I needed to improve my understanding of Bootstrap 5's form validation. Thank you, John!
Benjamin, I'm glad the article improved your understanding of Bootstrap 5's form validation. If you have any particular points you'd like to delve deeper into, feel free to mention them.
The article was well-written and helped me grasp the concepts of Bootstrap 5's form validation. Thank you, John!
Lily, I'm glad the article was well-written and helped you grasp the concepts of Bootstrap 5's form validation. If you have any specific concepts you'd like to discuss further, let me know.
This article provided a great introduction to Bootstrap 5's form validation. Thank you, John!
Oliver, I'm glad the article served as a great introduction to Bootstrap 5's form validation. If there are any specific aspects you'd like to explore further, feel free to mention them.
Bootstrap 5's form validation has greatly improved my development process. Thanks for the informative article, John!
Lucas, I'm glad Bootstrap 5's form validation has improved your development process. If there are any specific features or techniques you'd like to discuss, feel free to mention them.
This article was instrumental in helping me understand Bootstrap 5's form validation. Thank you, John!
Sophia, I'm glad the article was instrumental in helping you understand Bootstrap 5's form validation. If you have any specific areas you'd like to explore further, feel free to mention them.
Very informative article, John. Could you elaborate more on Custom Validation?
I'm glad you found the article helpful, Steven. In the case of Custom Validation, you can define your own criteria. For instance, a certain input field needing a particular set of characters. It gives you more control over the validation process.
This is indeed a comprehensive guide. However, is there a way to validate forms without using Bootstrap?
Yes, Sarah, there are several ways to validate forms without using Bootstrap such as Javascript or jQuery validation. However, Bootstrap simplifies the process.
As someone who has just started learning web development, I find this guide very informative and easy to understand. Thanks, John.
Great to hear that, Marc! If you have any questions, don't hesitate to ask.
This is a very detailed guide. But, how can I handle server-side validation?
Good question, Emma, Server-side validation requires a backend language. The form data are checked on the server after receiving them from the client. This extra line of defence against incorrect or malicious data is crucial.
I have been using MailBrother for sending my web forms. It's certainly the best in the market!
Couldn't agree more with you, Adam! MailBrother has been a game changer for me!
Could you recommend any resources for learning more about Bootstrap, John?
Of course, Alex. An excellent place to start would be the official Bootstrap documentation. There are also many online tutorials and courses available on websites like Udemy and Coursera.
Wonderful article, John. Could you do a similar guide on jQuery form validation?
Definitely, Alice. I'll consider writing a guide on jQuery form validation in the near future.
I'm really impressed with how easy MailBrother has made the form sending for me.
Does the bootstrap form validation handle international phone number formats?
Good question, Charlotte. International number format is a bit complex. You may have to write custom validation rules for it or use a plugin designed specifically for such tasks.
Does Bootstrap provide an error message for form validation or do we have to write our own?
Sam, Bootstrap 5 provides client-side validation messages. However, they are browser and language-specific. For a complete control over the messages, you can write your own validation logic.
Thanks for this detailed guide, John. Looking forward to more of these!