Four Ways to Make Text Case Insensitive in Web Development
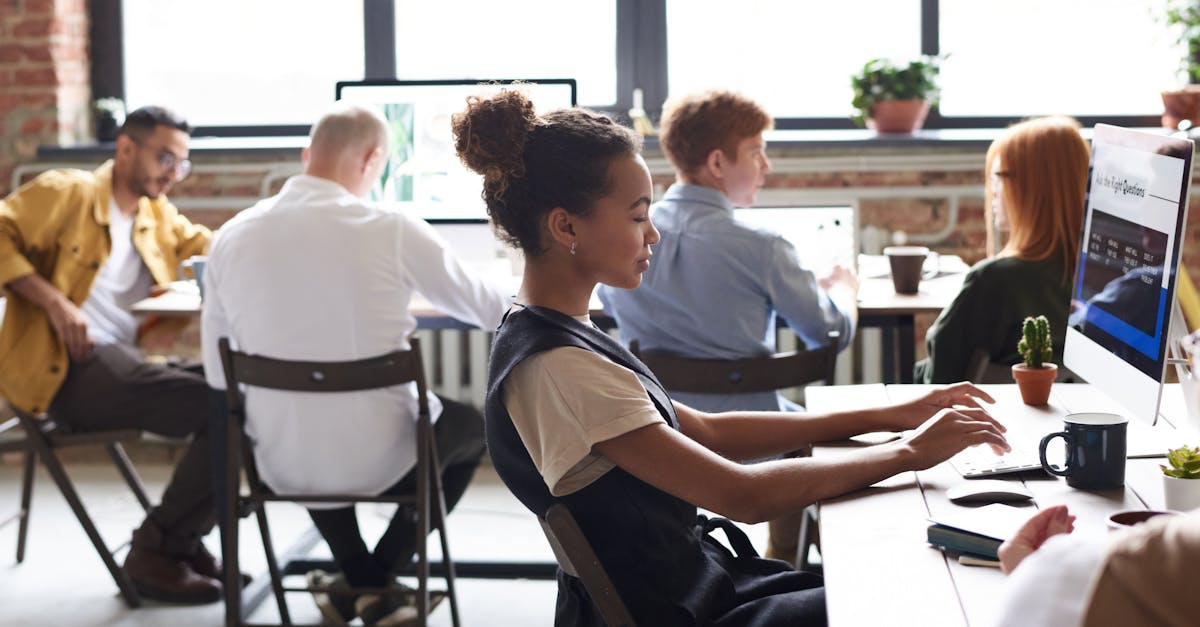
When working on web development projects, dealing with text input can sometimes be a challenge. One common issue is ensuring that the case of the text being input or compared doesn't affect its functionality. Fortunately, there are several ways to make text case insensitive in web development. In this article, we will explore four different methods to achieve this.
1. JavaScript toLowerCase() Method
One of the simplest ways to make text case insensitive is by utilizing the JavaScript method toLowerCase(). This method converts all characters in a string to lowercase. By converting both the input text and the text to be compared to lowercase, we can avoid issues that stem from differences in case.
Here's an example:
var inputText = "Hello";
var compareText = "hello";
if (inputText.toLowerCase() === compareText.toLowerCase()) {
alert("Texts are a match, regardless of case!");
} else {
alert("Texts do not match.");
}
In this example, the toLowerCase() method is applied to both the inputText and compareText variables, ensuring that the text comparison is case insensitive. The alert message will indicate whether the texts are a match or not.
2. CSS Text Transform
CSS also provides a way to make text case insensitive using the text-transform property. By setting the value to lowercase, we can ensure that the text input is always transformed to lowercase.
Here's an example:
input[type="text"] {
text-transform: lowercase;
}
In this example, any text entered into a text input field will automatically be transformed to lowercase. This ensures that the entered text is consistently in lowercase, making it case insensitive for comparison purposes.
3. Regular Expressions
Regular expressions can be a powerful tool in web development, including handling case insensitivity. By using the i flag in a regular expression pattern, we can specify that the matching process should be case insensitive.
Here's an example:
var inputText = "Hello";
var compareText = "hello";
var regex = new RegExp(compareText, "i");
if (regex.test(inputText)) {
alert("Texts are a match, regardless of case!");
} else {
alert("Texts do not match.");
}
In this example, the test() method of the regular expression object is used to check if the inputText matches the compareText, regardless of case. The i flag ensures that the matching is done in a case insensitive manner.
4. Server-Side Case Insensitivity
If you are storing and comparing text on the server-side, you can handle case insensitivity using server-side scripting languages or database functions. Many popular programming languages and databases offer built-in functions for handling case insensitivity, such as the ILIKE operator in PostgreSQL or the strcasecmp() function in PHP.
Here's an example using PHP:
$inputText = "Hello";
$compareText = "hello";
if (strcasecmp($inputText, $compareText) == 0) {
echo "Texts are a match, regardless of case!";
} else {
echo "Texts do not match.";
}
By utilizing server-side code, you can ensure that the text comparison is case insensitive, regardless of the user's browser or client-side behavior.
These four methods provide different approaches to making text case insensitive in web development. Choose the method that best suits your project's needs and ensure that your text input and comparisons are not affected by differences in case.
Comments:
Great article, Jonas! I've been struggling with this issue in my web development projects. Appreciate the tips.
Hi Michael! I'm glad you found the article helpful. Let me know if you have any questions or need further clarification.
Thanks for sharing these techniques, Jonas. It's always good to have case-insensitive options in web development.
Hi Jennifer! You're welcome. Indeed, having case-insensitive options can improve user experience and prevent potential issues. Let me know if you have any thoughts or additional suggestions.
I didn't know about these ways to make text case-insensitive. Thanks for sharing, Jonas!
Hi David! Glad I could introduce you to these techniques. Let me know if you have any questions or need further examples.
These methods are really handy! Thanks for the detailed explanations, Jonas.
Hi Sarah! I'm glad you found the explanations helpful. If you need further assistance or examples, don't hesitate to ask.
Thanks for this article, Jonas! These methods will definitely come in handy for my web projects.
Hi Alex! I'm glad you found the methods useful. If you have any questions or need further guidance, feel free to ask.
Thanks for this informative article, Jonas. Keep up the great work!
Hi Peter! Thank you for your kind words and support. I'm glad you found the article informative. If you have any suggestions for future topics, feel free to let me know.
Very useful techniques, Jonas. Thank you for sharing!
Hi Jessica! You're welcome. I'm glad you found the techniques useful. If you have any specific scenarios or questions, feel free to ask.
Great article, Jonas! Your explanations make it easy to understand. Thanks!
Hi Mark! Thank you for your feedback. I'm glad the explanations were clear. If you have any follow-up questions or need more examples, feel free to ask.
Thank you, Jonas! These techniques are very helpful for case-insensitive searches.
Hi Emily! You're welcome. I'm glad the techniques can assist you in case-insensitive searches. If you have any specific use cases or need assistance with implementation, feel free to ask.
Thanks for sharing these methods, Jonas. Really helpful!
Hi Robert! I'm glad you found the methods helpful. Let me know if you have any questions or if there's anything else I can assist you with.
These techniques are amazing, Jonas! Thank you for the insights.
Hi Karen! You're welcome. I'm glad you found the techniques amazing. If you have any specific scenarios or need further examples, feel free to ask.
Thank you, Jonas. This article has been very informative and will be useful in my projects.
Hi Daniel! I'm glad you found the article informative. If you have any questions or need further assistance during your projects, feel free to reach out.
These techniques are going to save me so much time! Thank you, Jonas!
Hi Laura! I'm glad the techniques will be time-savers for you. If you have any specific use cases or need assistance with implementation, feel free to ask.
Absolutely, Laura! the 'toLowerCase' method is a simple yet effective approach. It works for the majority of the cases.
Thanks for this informative post, Jonas. It's important to handle case-insensitive text properly.
Hi Steven! You're welcome. Indeed, handling case-insensitive text properly is crucial for a smooth user experience. Let me know if you have any thoughts or additional suggestions.
Great article, Jonas! These techniques will be very useful for my web development projects.
Hi Amanda! I'm glad you found the article great. If you have any questions or need further guidance during your web development projects, don't hesitate to ask.
So glad to hear you find our solutions helpful, Amanda! Thank you for your support.
These methods are exactly what I needed, Jonas. Thank you!
Hi Natalie! I'm glad the methods align perfectly with your needs. If you have any specific scenarios or need examples, feel free to ask.
Thank you for this valuable article, Jonas. It's definitely going to improve my web development projects.
Hi Matthew! You're welcome. I'm glad the article will contribute to the improvement of your web development projects. If you have any questions or need further assistance, feel free to reach out.
Fantastic article, Jonas! These techniques will definitely be applicable in my web projects.
Hi Sophia! I'm thrilled you found the article fantastic. If you have any questions or need assistance during your web projects, don't hesitate to ask.
Thank you for sharing these techniques, Jonas. Really appreciate it!
Hi Kevin! You're welcome. I'm glad you appreciate the techniques. If you have any thoughts or additional suggestions, feel free to share.
This article is a great resource, Jonas! Thanks for sharing your knowledge.
Hi Olivia! Thank you for your kind words. I'm glad you consider the article a great resource. If you have any specific use cases or need further examples, feel free to ask.
Thanks for explaining these techniques, Jonas. They will be very helpful in my projects.
Hi Jennifer! I'm glad you found the explanations helpful. If you have any questions or need further guidance in your projects, feel free to ask.
This article helped me understand the case-insensitive options better. Thanks, Jonas!
Hi Brian! I'm glad the article improved your understanding of case-insensitive options. If you have any follow-up questions or need more examples, feel free to ask.
Thank you, Jonas! Your explanations are clear and concise. This article has been a helpful read.
Hi Nicole! I appreciate your feedback. I'm glad you found the explanations clear and concise. If you have any additional questions or thoughts, feel free to share.
Fantastic article, Jonas! These techniques will definitely be helpful in my current project.
Hi Joshua! I'm thrilled you found the article fantastic. If you encounter any challenges or need assistance during your current project, don't hesitate to reach out.
Thanks for sharing this knowledge, Jonas. These methods will be very useful in my future projects.
Hi Eric! You're welcome. I'm glad you found the methods valuable. If you have any questions or need further guidance for future projects, feel free to ask.
Thank you, Jonas! This article has provided great insights into handling case-insensitive text.
Hi Rachel! I appreciate your feedback. I'm glad the article provided great insights into handling case-insensitive text. If you have any specific scenarios or questions, feel free to ask.
Thanks for sharing these techniques, Jonas. They will be very useful in my web development projects.
Hi Matthew! I'm glad you found the techniques useful for your web development projects. If you have any questions or need further assistance, feel free to reach out.
Great article, Jonas! These methods will definitely come in handy.
Hi Lauren! I'm glad you found the article great. If you need any assistance or have any questions about the methods, feel free to ask.
Thanks for sharing these techniques, Jonas. They will be very useful in my future projects.
Hi Brandon! You're welcome. I'm glad you found the techniques useful for your future projects. If you have any specific scenarios or need examples, feel free to ask.
Thank you for this informative article, Jonas. It has expanded my knowledge in web development.
Hi Emily! Thank you for your kind words. I'm glad the article has expanded your knowledge in web development. If you have any further questions or need more insights, feel free to reach out.
These techniques are amazing, Jonas. Thank you for sharing!
Hi Justin! I'm glad you found the techniques amazing. If you have any specific scenarios or questions, feel free to ask.
Thank you, Jonas! This article has been very helpful for me.
Hi Vanessa! I'm glad the article has been helpful. If you need any further assistance or have any questions, feel free to ask.
This article is a great resource, Jonas. Thanks for sharing your expertise.
Hi Catherine! I appreciate your kind words. I'm glad you consider the article a great resource. If you have any specific use cases or need further examples, feel free to ask.
Thanks for sharing this knowledge, Jonas. It will definitely be useful in my future projects.
Hi Henry! You're welcome. I'm glad you found the knowledge valuable for your future projects. If you have any questions or need further guidance, feel free to ask.
Thank you, Jonas! These techniques are exactly what I needed.
Hi Samantha! I'm glad the techniques align perfectly with your needs. If you have any specific scenarios or questions, feel free to ask.
This article is excellent, Jonas. It provides clear guidance on an essential topic.
Hi David! Thank you for your positive feedback. I'm glad the article provides clear guidance on an important topic. If you have any thoughts or additional suggestions, feel free to share.
Thanks for sharing these techniques, Jonas. They will be very handy!
Hi Martin! I'm glad you found the techniques handy. Let me know if you have any questions or need further assistance.
Thank you, Jonas! This article has been very informative for me.
Hi Megan! I'm glad the article has been informative for you. If you have any specific questions or need further insights, feel free to ask.
Fantastic article, Jonas! These techniques will be really helpful in my projects.
Hi John! I'm thrilled you found the article fantastic. If you encounter any challenges or need assistance in your projects, don't hesitate to reach out.
Thank you for explaining these techniques, Jonas. They will be very useful in my web projects.
Hi Victoria! You're welcome. I'm glad you found the explanations helpful. If you have any questions or need further guidance in your web projects, feel free to ask.
Thanks, Ronald! Regular expressions can be utilized by using flags. The 'i' flag makes the entire expression case-insensitive. Is there anything specific you'd like to know?
Good point, Chris. CSS method, indeed, due to its dependency on browser implementations, may behave differently across various web browsers. Testing is vital here.
Agree, Naomi. Turning both strings to the same case can be quite reliable in most conditions. It is the method I personally use the most.
Logan, glad you found something new from the article. Practice is the best way to learn!
Thanks, Ian. Performance-wise, differences are usually negligible in general use cases. But, in heavy data processing, 'toLowerCase' and 'toUpperCase' may perform a bit better.
Thank you, Eleanor! I'm glad that this article helps you. Remember, practice is key in web development. Keep coding, keep improving!
Well pointed out, Samuel! The 'locales' parameter indeed helps in providing language-specific sorting.
Great article Jonas. Well detailed and highly informative. However, I have a question about the regex method. How can it handle special characters in the text?
Tried the convert to uppercase method, it worked like a charm. Thanks, Jonas!